本文主要介绍了几种在数据展示时,常用的画图的方法
ax画图常用的参数
fig,ax: 画板,用法:fig, ax = plt.subplots(figsize=(10, 5))
figsize: 表示图像大小的元组
sharex, sharey:x(y)轴共享,用法:sharex=True
title: 标题
legend: 添加图例,用法:ax.legend([‘kind1’,’kind2’])
xticks,yticks: x(y)轴的显示备注,用法:ax.set_yticks(‘人数’)
xticklabels: x轴标签内容,用法:ax.set_xticklabels([‘kind1’,’kind2’])
xlim,ylim: x(y)轴刻度的上下限,用法 ax.set_xlim(0, 1)
fontsize: 字体大小
colormap: 颜色
alpha: 透明度
sort_columns: 按照字母顺序绘制,默认使用当前列顺序
grid: 轴网格线,默认打开
bins: 柱子的数量
matplotlib中的常用图形
line: 线形图
bar: 垂直柱状图,stacked=True时是堆积柱状图
barh: 水平柱状图
kde: 核密度估计图
hist: 频率分布直方图
area: 面积图
matplotlib画图
导入需要的包
|
|
|
|
kind | num1 | num2 | |
---|---|---|---|
0 | kind1 | 1 | 0.4 |
1 | kind2 | 2 | 0.5 |
2 | kind3 | 3 | 0.6 |
柱状图
|
|
柱形图结果
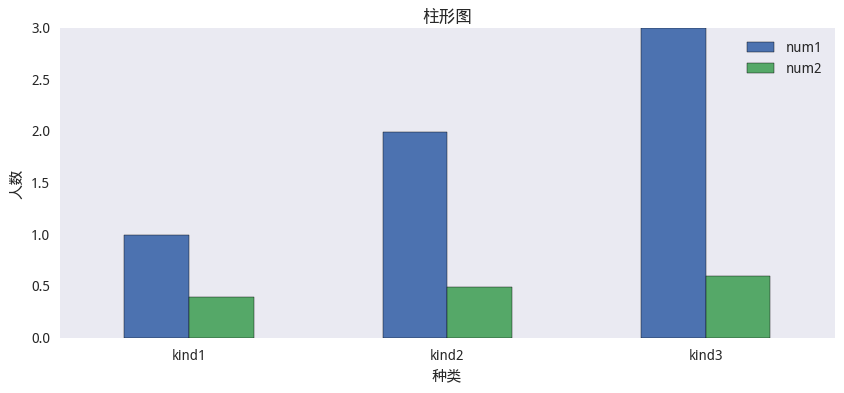
带数字的柱状图,并把值改成百分比显示
- 此处定义了一个函数,目的是将数字转化成百分比形式
|
|
|
|
百分比柱状图结果
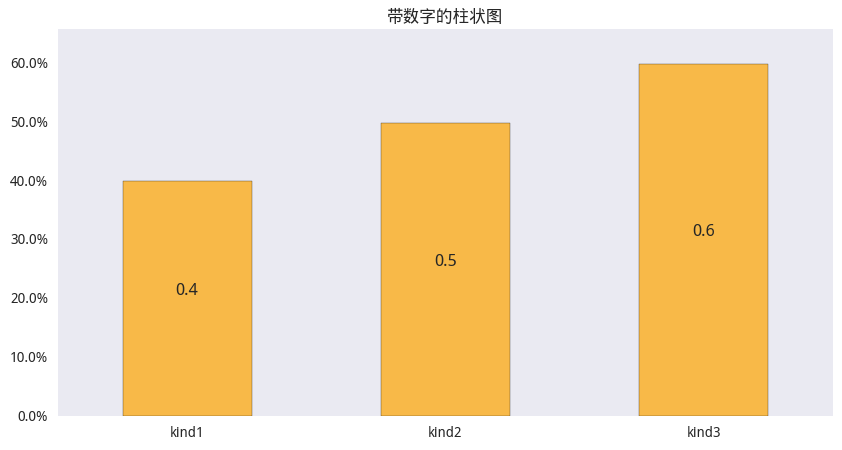
线形图
|
|
|
|
线形图结果
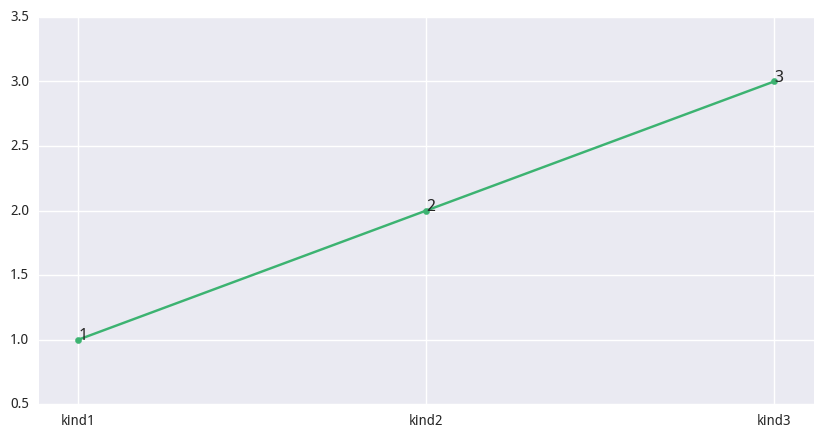
两类占比对比柱形图
- True和False的值相加为1
|
|
|
|
kind | True | False | |
---|---|---|---|
0 | a | 0.9 | 0.1 |
1 | b | 0.1 | 0.9 |
2 | c | 0.5 | 0.5 |
|
|
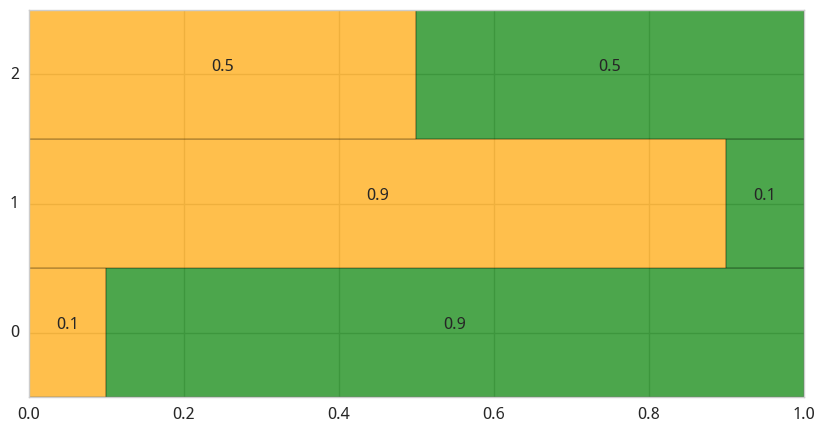